Size
Concept
Establishing the size of a picture is sometimes needed for complex layout, and the Size algebra provides this.
Bounding Box
The core method, boundingBox
, gets the BoundingBox of a picture.
import doodle.core.*
import doodle.java2d.*
import doodle.syntax.all.*
val circle =
Picture
.circle(100)
.strokeColor(Color.midnightBlue)
val circleBoundingBox: Picture[BoundingBox] =
circle.boundingBox
As the type annotation suggests, this returns a Picture[BoundingBox]
rather than a BoundingBox
.
In other words, the BoundingBox
will be calculated when the Picture
is rendered and is not immediately available.
This is necessary because the size may depend on backend-specific calculations, such as the space occupied by text.
To make use of the BoundingBox
in the creation of a picture we can use the flatMap
method on Picture
.
val boundingBox =
circleBoundingBox.flatMap(bb =>
Picture
.roundedRectangle(bb.width, bb.height, 3.0)
.strokeColor(Color.hotPink)
.strokeWidth(3.0)
)
// boundingBox: Picture[FromGifBase64 & Basic & Bitmap & FromBufferedImage & FromPngBase64 & FromJpgBase64, Unit] = doodle.algebra.Picture$$anon$1@73d513d2
val picture = circle.on(boundingBox)
// picture: Picture[Bitmap & FromBufferedImage & FromPngBase64 & FromGifBase64 & Basic & FromJpgBase64, Unit] = doodle.syntax.LayoutSyntax$$anon$1@18ab50f9
This produces the picture shown below.
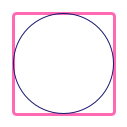
Other Methods
The Size
algebra defines a few utilities derived from the boundingBox
method:
width
gets the height of the enclosing bounding box, as aDouble
;height
gets the height of the enclosing bounding box, as aDouble
; andsize
gets the width and height of the enclosing bounding box, as a tuple(Double, Double)
.
Implementation
The Size algebra supports all the features described above. Image does not currently support these operations.